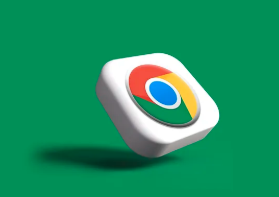
Selenium ChromeDriver is important for running automated tests on Google Chrome using Selenium WebDriver. It essentially works as a link between the Selenium test scripts and the Chrome web browser, allowing both to communicate with each other and automating the process. But Using ChromeDriver alone is not enough. You can optimize the ChromeDriver configuration to speed up and make the test run more stable.
In this article, we will discuss what is Selenium ChromeDriver, the functionality of ChromeDriver with details on the ChromeDriver features set, the best practices that can be followed to enable faster, more reliable test execution, avoid common issues and how they can be fixed. Regardless of your status as a new or competent automation tester, this approach will guide you on how to use ChromeDriver to its fullest capacity as a part of your Selenium test automation plan.
What Is Selenium Chrome Driver?
ChromeDriver is a standalone server that connects with Google Chrome for running your Selenium WebDriver tests. It is a Chrome-specific implementation of WebDriver, which connects testers and allows them to automatically perform user gestures in Chrome. A chromeDriver is an intermediary for crawling (Selenium WebDriver communication with the Chrome browser). Without ChromeDriver, the Selenium WebDriver cannot crawl the Chrome browser, the tool essential for crawling the browser.
Key Functions of ChromeDriver
ChromeDriver is the main component of Selenium WebDriver that allows automated testing in Google Chrome. The ChromeDriver acts as an intermediary between Selenium scripts and the browser, taking your WebDriver commands and translating them into actions in Chrome.
The main functionalities it provides are launching and controlling browser sessions, manipulating web elements, executing JavaScript, managing cookies and configuring browser settings. Utilizing the powers of ChromeDriver, testers can automate elaborate workflows, witness UI interactions and verify the smooth functioning of web applications.
- Handling Browser Sessions: ChromeDriver starts and manages a Chrome browser session for test execution.
- Working with Web Elements: It allows Selenium command to work with web elements, e.g., clicking buttons, entering data in forms, and navigating through pages.
- Running JavaScript: ChromeDriver also allows you to run JavaScript commands from within the browser.
- Manage Chrome Preferences: You can modify chrome settings like disabling pop-ups and parameters, creating a custom user agent, etc.
Setting ChromeDriver for Selenium
Installing ChromeDriver is the first step in executing automated tests with Selenium WebDriver. However, correct installation and configuration allow testers to execute their tests without errors in communication between the browser and the driver. We will cover a step-by-step guide for downloading, installing and setting up ChromeDriver to work with Selenium in this section.
Step 1: Download and Set Up ChromeDriver
- Check Chrome version: Go to chrome://settings/help to see your version.
- Download ChromeDriver: Go to the official ChromeDriver download page and download the appropriate version for your Chrome browser.
- Extract and Configure: Unzip the downloaded and place the chromedriver exe (or its equivalent for macOS/Linux) file into a folder.
- Configuring Path: Either add ChromeDriver location to system environment variables or specify it directly in your test script.
Step 1: Configure ChromeDriver in Selenium
Once you have downloaded the ChromeDriver, you will have to configure it in your Selenium webdriver.
The following lines of code need to be included in your test script to launch Chrome using Selenium WebDriver:
System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver”);
WebDriver driver = new ChromeDriver();
driver.get(“https://www.example.com”);
Making ChromeDriver Run Fast And Stable Tests
Using Selenium for automated tests needs to be fast and stable to run and validate the essential test harmonics. If ChromeDriver is not optimized properly, it can result in slow test execution or flaky tests, which can indirectly lead to wasted time and resources. By optimizing ChromeDriver configurations, following best practices, and identifying performance bottlenecks, testers have the power to improve execution speed and reduce the need for test re-runs.
In the following section, we look at key techniques for ChromeDriver performance optimization, including headless test execution, disabling features of the Chrome browser not used for the test, employing better locator strategies, and how to control outsourcing or interruption of browser sessions. These optimizations make sure that your Selenium tests execute seamlessly, allowing faster feedback loops and more consistent results.
Run Chrome in Headless Mode
The headless way of running Chrome reduces the time to test execution. Use the following command:
ChromeOptions options = new ChromeOptions();
options. addArguments(“–headless”);
driver = new ChromeDriver(options);
This minimizes the cost of rendering the UI of the browser, which gives a boost in execution speed.
Disable Unnecessary Features
Chrome itself runs many default features that could potentially decrease automation speed. This can boost performance by disabling them.
options. add arguments in chrome options –disable-extensions
options. addArguments(“–disable-infobars”);
options. addArguments(“–no-sandbox”);
options. addArguments(“–disable-dev-shm-usage”)}
These settings further minimize resource utilization, particularly on CI/CD pipelines.
Explicit Waits Over Implicit Waits
OImplicit waits slow down the tests when the element loads slower than the given parameter. Instead, use explicit waits:
WebDriverWait wait = new WebDriverWait(driver, Duration. ofSeconds(10));
wait. until(ExpectedConditions. elementToBeClickable(By. id(“submit-button”))). click();
By doing this, interaction with elements is possible only when the elements are ready to be interacted with.
Optimize Locator Strategies
What you can do to improve test stability is use efficient locators:
- Prefer By. id or By. name over By. xpath or By. cssSelector when possible.
- Do not use dynamic XPath expressions that change too often.
Close Unused Tabs and Processes
Clear the Chrome session, leaving just the tabs you want to use and empty the caches:
driver. manage(). deleteAllCookies();
ArrayList tabs=new ArrayList(driver. getWindowHandles());
for (int i = 1; i < tabs. size(); i++) {
driver. switchTo(). window(tabs. get(i)). close();
}
driver. switchTo(). window(tabs. get(0));
Common Issues and Solutions
Selenium ChromeDriver is a great tool for automating tests, but it comes with challenges. These issues, such as version mismatches, session failures, browser crashes, and element interaction errors, are proving to be real problems for the testers that need to be resolved and they impact test execution and reliability of testing.
Since the automation framework is a very critical component, it is important to recognize these common problems and know how to solve them. In this segment, we will walk through the common issues faced by ChromeDriver users and share workarounds to fix, allowing you to run your tests smoothly and in a timely fashion.
ChromeDriver Version Mismatch
Problem: ChromeDriver does not open because the version is not compatible. Problem: ChromeDriver is not supporting your Chrome version
Solution: Always Download ChromeDriver with the Chrome browser version
SessionNotCreatedException: Message: session not created
Problem: The browser session does not get initialized.
Solution: Make sure ChromeDriver and Chrome are on the latest versions. Verify that the path to ChromeDriver is set accurately.
Element Click Intercepted Error
Issue: When Selenium clicks on an element, but the element is overlapped by another element.
Solution: Use explicit waits to ensure the element is interactable.
JavascriptExecutor js = (JavascriptExecutor) driver;
js. executeScript(“arguments[0]. scrollIntoView();”, element);
Chrome locks up or crashes while you take tests
Issue: The test gets hung due to resource exhaustion. Solution:
Solution: Run tests in headless mode. This will avoid memory issues: –no-sandbox –disable-dev-shm-usage.
Integrating ChromeDriver in your CI/CD Pipeline
Seamlessly executing automated testing for every code change through integration of Selenium ChromeDriver in a Continuous Integration/Continuous Deployment (CI/CD) pipeline makes continuous delivery of high-quality software possible. Yet, badly configured tests can become flaky, slow, or fail in dynamic environments.
To speed up the execution of tests using ChromeDriver, other practices need to be optimized: ChromeDriver settings, headless execution, dependencies, and parallelizing tests. In this post, we wanted to look into some of the best practices for using ChromeDriver in CI/CD, providing teams with an opportunity to achieve fast feedback loops, test stability, as well as optimize their deployment process.
- Use Docker: Run Ubuntu Selenium Docker containers to make sure your environment remains consistent.
- Execute Tests in Parallel: Employ Selenium Grid or TestNG to run tests simultaneously and minimize execution time.
- Remote WebDriver: split the execution on Selenium Grid to reduce the load.
- Make Sure ChromeDriver is Up to Date: Compatibility issues can arise due to outdated ChromeDriver.
- Use Cloud testing platform: Cloud testing platforms like LambdaTestintegrates seamlessly with CI/CD pipelines, enabling automated cross-browser testing at scale. By incorporating LambdaTest into your CI/CD workflow, you can ensure that every code change is tested across multiple browsers, devices, and operating systems before deployment.
LambdaTest is an AI-native test orchestration and execution platform that enables QA engineers to perform manual and automated testing at scale across 5000+ environments, including real browsers, devices, and operating systems. It supports parallel test execution, AI-powered test analytics, and cloud-based debugging tools, ensuring faster, more reliable, and efficient testing.
With integrations for Selenium, Appium, Playwright, JUnit, and more, LambdaTest helps teams achieve seamless cross-browser, mobile, and performance testing while accelerating release cycles.
Conclusion
Selenium ChromeDriver is a great tool to automate tests on Google Chrome but to be able to run there in an efficient/deterministic manner, we will configure the default values to run faster and less buggy tests. Utilizing headless mode, disabling unwanted features, implementing explicit waits and following best practices to boost your test automation performance. Also, proactively troubleshooting common issues can lead to a smooth test execution.
Using these techniques, teams will create strong automation frameworks with less flakiness, providing faster cycles of software delivery and better-quality applications. Happy testing!
RECOMMENDED ARTICLES
The Ultimate Guide to MyLiberla.com Blog
Software Updates Durostech: Everything You Need to Know
SSIS 950: Comprehensive Guide to SQL Server Integration Services 950