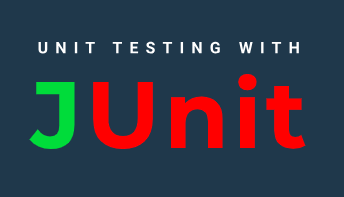
Automated testing replaces manual testing and is a key component of modern software development, reducing the amount of manual work required to ensure the reliability, stability, and maintainability of code. JUnit is probably the most used Java framework for unit testing. Writing JUnit tests effectively can enable developers to spot bugs early, avoid regressions and ensure better coding quality.
This article will cover the basics of JUnit testing, tips for good practices and advanced methods for writing tests that will keep your software safe from disasters.
What Is JUnit?
JUnit is an open-source testing framework for Java that is very popular. It offers you an organized approach for writing and executing automated tests that check whether the different units of your code (functions, classes, or modules) behave as required. It is easy to use with build tools such as Maven and Gradle and integrates well with CI/CD pipelines.
Key Features of JUnit:
JUnit is a popular unit testing framework that makes it easier to test individual units of code in Java. It is a widely used testing framework for Java applications due to its powerful features. JUnit also includes support for parameterized tests, exception testing, and easy integration with build tools and CI/CD pipelines. In this chapter, we will discuss how JUnit provides some sample test features that can speed up the process of testing and writing better code.
- Annotations to organize the test cases in an effective fashion.
- Assertions for verification to compare expected values to actual output.
- Test lifecycle management to manage the creation/destruction of test environments.
- These allow you to make parameterized tests for multiple scenarios to avoid writing duplicate test cases.
- Most modern test tools provide integration with IDEs and build tools, making it easy to run tests during development.
JUnit Architecture
JUnit uses a good architecture to coordinate unit tests in a simple, organized way. You are on the modular architecture which is made up of multiple important components collaborating to help automate tests.
Test Runner
Test Runner runs test cases and reports results. It has the ability to identify test methods and run them independently, giving feedback as to whether the test has passed or failed. JUnit implements different test runners, such as the default one and specific test runners created by developers.
Test Fixtures
A Test Fixture makes sure that every test has a clean and controlled environment. JUnit offers a series of lifecycle annotations, like @BeforeEach and @AfterEach, to handle setup and cleanup of test conditions, creating dependencies between test executions.
Test Cases
Test cases are individual methods marked with @Test that include assertions to check for expected behavior. You are given trains of data till October 2023.
Assertions
Assertions, which are contained in the Assert class in JUnit, allow us to validate that the results of our tests are correct. These commonly include checking for equality, checking for conditions, or catching exceptions; to ensure that the output you received actually matched what you expected.
Test Suites
This will help us to launch one of more tests as a whole, and a Test Suite serves as a container for one or more individual tests. This helps developers group related tests semantically and execute them in groups for thorough validation of related functionality.
Annotations
Annotations are heavily used in JUnit to specify and manage test execution. Some key annotations include:
- @Test – Indicates that a method is a test case.
- @BeforeEach / @AfterEach – Sets up and tears down resources for every test.
- @BeforeAll / @AfterAll – Runs a setup and teardown once before and after all tests in a class.
- @Disabled — Ignores a test case for some time.
Exception Handling and Parameterized Tests
JUnit additionally includes functionality for testing exceptions and executing parameterized tests, permitting developers to succinctly and accurately validate numerous input situations.
JUnit’s architecture, by incorporating these fundamental building blocks, provides a structured and reliable framework for unit testing, which is essential for Java developers aiming for high-quality software.
Setting Up JUnit
Note that JUnit has to be added to your project to be able to use it. You can add the dependencies in your configuration files based on your build tool. After JUnit is installed, you can write test cases to ensure your code is correct.
Writing Effective JUnit Tests
A well-written test validates expected behavior as well as captures potential bugs well before they make it into production. This can be accomplished by adhering to best practices like using meaningful names for our tests, following the Arrange-Act-Assert (AAA) testing pattern, and ensuring that we cover all possible scenarios, even the edge cases. Properly written cases are easier to read and team does not have to break a sweat finding the issues during their testing.
This section will discuss some important tips behind writing JUnit tests to save cost and build great value over time to your software products.
Follow the AAA Pattern
While writing test cases, use the Arrange-Act-Assert approach to keep your tests clear. This technique consists in preparing the contextual test conditions (Arrange), running the functionality to be validated (Act) and asserting the resulting output according to expectations (Assert). Never fails to provide a well-structured & readable test.
Use Meaningful Test Names
A X Test Name, should tell you exactly what the X Test case is verifying. Descriptive names for tests help understand the failure, what failed and how. Don’t use names like test1() — instead describe the expected behavior.
Test Edge Cases
Writing tests for common paths is important, but edge case validation is just as important. So there are some values such as boundary values, negative input, null values, and extreme conditions. Test edge cases to make sure your application is working under all possible situations.
Advanced JUnit Techniques
Indeed, as you become more familiar with the JUnit framework, understanding advanced Testing techniques will allow you to create optimized, scalable, and maintainable test cases. Additional features like parameterized tests, exception testing, and test stubbing allow developers to improve code coverage while making test writing more convenient.
This not only decreases redundancy but also improves the milestones quality as it can simulate real-world scenarios effectively. In this part, we’ll delve into some advanced JUnit techniques that can elevate your testing skills and help you build solid and high-performing applications.
Using Parameterized Tests
Annotated tests are great to avoid writing the same kind of test multiple times. With this solution, verbosity is lowered and coverage of the tests is better done.
Testing Exceptions
Testing is not just about making sure your application runs, but also about handling exceptions correctly. Ensuring expected exceptions are thrown under certain circumstances guarantees your error handling mechanisms work as they should.
Mockito – Mocking Dependencies
Using a mocking framework (e.g., Mockito) helps isolate the unit under test when testing components that have external dependencies. Mocking enables to simulate responses from external services with the internal code only, thus making the tests more stable and independent.
JUnit Testing Best Practices
JUnit testing is essential to ensure the functionality and performance of Java applications. Writing effective tests is more than confirming the expected output. This is to make sure test cases are structured, well maintained and “able to catch potential problems early in the development cycle”.
Following these rules helps you keep tests independent and readable, use assertions effectively, and integrate with CI/CD pipelines, all of which improve the quality of your software and speed up your debugging.
In this part, we will discuss fundamental best practices that should help you write solid JUnit tests and avoid common mistakes.
- Test Independence – Write tests in such a way that they do not communicate via shared state with other tests. This avoids unintended dependencies and is also easier to debug.
- Use Setup and Teardown Methods – Lifecycle methods such as @BeforeEach and @AfterEach are useful to set up and tear down resources for each test.
- Do not overuse mocks – Mocks can come in handy, but excessive use could lead to more complex and unrealistic tests. Use mocks and other dependencies for unit tests sparingly.
- Execute Tests Regularly – Incorporate tests into CI/CD to identify issues early and ensure code stability.
- Use Assertions Wisely – Assertions are used to verify the correctness of test cases. Use comprehensive assertions to test the variety of expected behavior.
- Use cloud testing platforms – Cloud-based AI native testing platforms like LambdaTest enable you to perform manual and automated tests at scale across 5000+ real browsers, devices, and OS environments.
With features like parallel execution, AI-native test insights, and seamless CI/CD integrations, LambdaTest helps teams enhance test coverage, accelerate execution, and ensure cross-browser compatibility without infrastructure constraints.
Common Mistakes to Avoid
So, while JUnit testing improves code quality and prevents regressions, badly written tests can give false results and slow down the development process. Some developers make some very common mistakes — leaving edge cases, writing brittle tests that break on changes, mock abuse, bad test performance, etc. These pitfalls can cause your test suite to be less effective and generate false positives or fail to detect defects. In this section, quotes will focus on the most common mistakes in JUnit testing and solutions on how to avoid them, keeping your tests reliable, maintainable, and performant.
- Not Testing Edge Cases – Not testing boundary scenarios may result in unexpected failures.
- Fragile Tests – Tests that have to be updated every time the code changes slightly are not reliable and are tedious.
- Performance Issues – If you have a large battery of tests, this should give itself to be optimized for execution speed to provide you with rapid feedback.
- Skipping Integration Tests – Unit tests alone don’t cut it; supplement those with integration and system tests to ensure end-to-end functionality.
Conclusion
JUnit is a powerful unit testing framework that enables Java developers to write reliable and maintainable tests. However, adopting best practices and advanced techniques and avoiding common pitfalls is essential to ensuring tests effectively safeguard your software.
For web application testing, integrating JUnit with Selenium and ChromeDriver allows for automated browser testing, ensuring web apps function correctly across different environments. Selenium ChromeDriver provides a direct connection between Selenium WebDriver and the Chrome browser, enabling fast, reliable test execution.
By incorporating JUnit and Selenium ChromeDriver into your development workflow, you can catch bugs early, enhance code quality, and ensure software stability—saving time, effort, and costly production issues. Start writing better tests today and build software that truly lasts!
RECOMMENDED ARTICLES
iCryptoAI.com Innovation: Transforming Cryptocurrency Trading with AI
Artofzio: The Ultimate Guide to Revolutionizing Digital Art
The Ultimate Guide to adsy.pw/hb3: Boost SEO & Brand Visibility